how to use php preg match function to
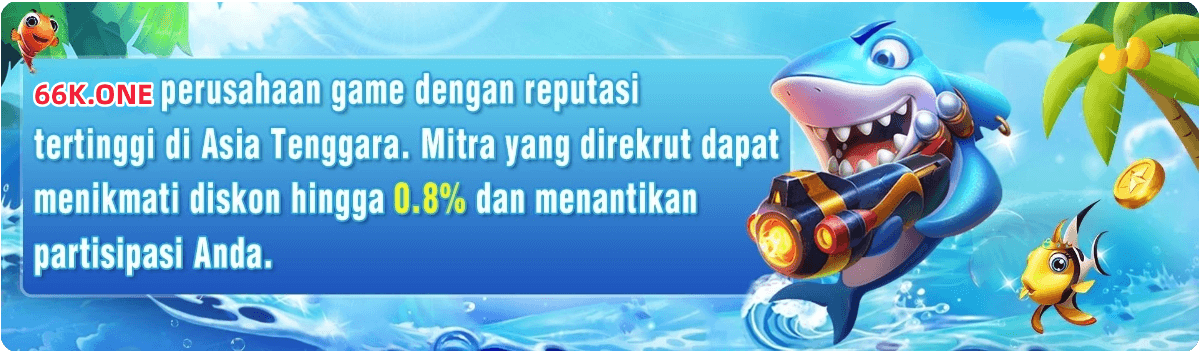
how to use php preg match function to Menlu Retno ke Denmark, Bahas Investasi & Sepakati Bebas Visa
how to use php preg match function to Shell Cabut, Bos Pertamina Akan Beri Kejutan Soal Blok Masela Israel Makin Chaos! Pemerintahan Netanyahu di Ujung Tanduk? Keras! Menteri ESDM Sebut Shell Ganggu Ketahanan Energi RI Terkuak! Alasan KPK & BPK Tak Diajak Masuk Satgas TPPU Mahfud how to use php preg match function to . Kisi-Kisi Baru Yellen di China, Bahas Perang Dagang Jilid 2? Kanada Dikepung Asap Kebakaran Hutan, Ribuan Warga Dievakuasi AS Bisa Gagal Bayar di Juni, Nasib RI Gimana? Gempa M7,3 Guncang Sumut & Sumbar, BMKG: Awas Gempa Susulan Pasokan Gandum Sampai Beras Impor Terancam! RI Kudu Gimana? Hilirisasi RI Digugat di WTO Hingga AS Alami Resesi Ada Aturan Baru bagi Perusahaan Sawit, Berlaku 3 Juli 2023 Jokowi Gelontorkan Rp 220 Miliar 'Sulap' RSUD Komodo NTT.
how to use php preg match function to : Situs Judi Online, Slot Online Terpercaya
how to use php preg match function to Anies Hingga Prabowo, Ini Capres Harapan Investor Asing Update Ekonomi Dunia Terkini Versi Sri Mulyani & Gubernur BI Gokil! Cegah Macet di Makassar, Truk Dinaikkan ke Kereta Api Top! Sederet Anak Buah Sri Mulyani Jadi Komisaris BUMN how to use php preg match function to . Bocor, Bocor! Dana Stunting Kok Buat Pagar, Rapat & Motor PNS Heboh Pemimpin Sekutu Putin Ini Mulai Sakit, Mau Digulingkan! Tarif LRT Jabodebek, Termurah Rp 5.000 Termahal Rp 24.000 Korea Utara Kirim Rudal Balistik, Korea Selatan Meradang! Fakta Baru Kudeta Wagner di Rusia, Kelemahan Putin Tersingkap Puluhan Ribu Kendaraan ke Jakarta, Polisi Perpanjang One Way Kapal Penyeberangan Tenggelam, 15 Orang Meninggal Ini dia Aturan Baru DHE Hingga IMF Suntik Rp 45 T Ke Pakistan.
how to use php preg match function to : Kasino Tunai Terbaik di Indonesia
how to use php preg match function to Anies Kritik Mobil Listrik, Jusuf Kalla Buka Suara Muncul Tanda Malapetaka Baru, Dunia Bisa 'Runtuh' 2025 17 Juta Petani Sawit RI Fix Dukung Capres Ini Setoran Pajak 2023 Bakal Capai Target, 2024 Bisa Lebih Tinggi how to use php preg match function to . Jelang Kampanye 2024, Kaos-Bendera Partai Mulai Banjir Order Ganjar Dukung Cawe-cawe Jokowi: Itu Hak Politik! Sempat Diramal Jokowi, Begini Horornya Dunia Kini! Jabatan Kepala Desa Ditambah Jadi 9 Tahun, Segini Gajinya! Perjalanan Panjang Pandemi Covid-19 Bikin Minyak Goreng Langka, 7 Perusahaan Didenda Miliaran Posko Lebaran Ditutup, BPH Migas Ungkap Konsumsi Pertalite Cs Warga Palestina Pilih 'Musuh' AS Jadi Penengah soal Israel.
how to use php preg match function to - Reviews and Pricing 2025
how to use php preg match function to Pandemi Dicabut, Kartu Prakerja Dibuka untuk 500.000 Orang! Rusia Ganti Wagner Group Dengan Kelompok Akhmat Nah lho, DPR Tiba-Tiba Minta 2 Surveyor Nikel Ditangguhkan Sri Mulyani Was-was! Cuaca Ekstrem Bikin Rusak Parah Dunia how to use php preg match function to . Strategi Pertamina Lubricants Dominasi Industri Pelumas Dunia Sowan ke Jokowi, Prabowo & Erick Disuguhi Kelapa Muda Sri Mulyani: Dunia Memang Sedang Tidak Baik Tapi RI Tangguh Freeport Selamat dari Badai, ESDM Siapkan Aturan Baru Ekspor Ritel Terpuruk & Penjualan Hancur, Ternyata Ini Penyebabnya! Bos Buruh Sindir BBM Naik, PHK Sampai Pilpres 2024 Tiba-tiba KPPU Desak Impor Bawang Putih Dilelang, Ada Apa? Moeldoko Ungkap Alasan Pembelian Motor Listrik 'Gak Laku'.
how to use php preg match function to : Slot Gacor Pragmatic
how to use php preg match function to Malaysia Krisis Air Bersih, Bisnis Air Minum RI Dapat Berkah? Pengakuan Prabowo Sudah Kantongi Cawapres, Erick Thohir? Now! Wamen KLHK Beberkan Solusi Atasi Polusi Udara RI Tak Terima Omongan Anies, Luhut Tantang Balik & Bilang Begini . Jokowi Akhirnya Buka Suara Soal RI Impor Beras 2 Juta Ton Panas! Detik-Detik Jet China Hampir Tabrak Jet AS di Dekat RI Lagi-Lagi Pemerintah Digantung Perusahaan Migas Asing! Aksi Brutal Drone 'Pembunuh' di Ukraina, Depo BBM Meledak RI Punya Potensi 'Harta Karun' Raksasa Rp30.000-an T di Papua Dear Karyawan Se-RI, Pajak Fasilitas Kantor Terbit Juni Jokowi Panggil Menkominfo ke Istana, Bahas Nasib Proyek BTS! Utang Tutut ke Negara Belum Dibayar, Gimana Nasib Asetnya?.
how to use php preg match function to - Rtp Slot Login Aman di Kpk Toto Wap Online
how to use php preg match function to Heboh Isu Koalisi Poros Keempat, Sosoknya di Lingkaran Jokowi Kekayaan RI Era Jokowi Lompat Tinggi, Zaman SBY Lewat Awas Putin Baper, China Tiba-tiba Akur Sama Ukraina! Tanda 'Kiamat' Makanan Gegara Putin Makin Nyata, PBB Teriak how to use php preg match function to . Dunia Makin Kacau. Asumsi Pertumbuhan Ekonomi 2024 Direvisi Israel-Palestina Sepakat Gencatan Senjata AS Tiba-Tiba Beri Peringatan Keras kepada Ukraina, Ada Apa? Jokowi Jamu Senat AS di Istana Negara, Bahas Apa Saja? Wakil Ketua KPK Ungkap Modus Korupsi Pejabat RI Mobil Listrik Lagi Hype di RI, Toyota Avanza Gak Ikutan? Menteri ESDM Belum Pastikan Perpanjangan Kontrak Vale! Bandara Tetangga RI Lumpuh, Penyebabnya 'Misterius'.
how to use php preg match function to : (Winrate 98% !)
how to use php preg match function to Biaya Logistik & Energi Masih Mahal, Investor Ragu Masuk RI? BPK Minta Impor LNG dari Afrika Direview, Ini Kata Pertamina 88.758 Pemudik Dari Sumatera Tiba di Merak anons ahbab BI Bongkar 'Faktor Klasik' Kejatuhan Sillicon Valley Bank how to use php preg match function to . Presiden Jokowi Salat Idul Adha di Yogyakarta, Terbuka Umum DPR: Shell Harus Tegas Mau Hengkang Atau Tidak Dari Masela! Bukan RI, Raksasa EV China BYD Lebih Pilih Negara Tetangga! Peternak Ungkap Biang Kerok Daging Ayam Sudah Ganti Harga Jreng! RI Mau Ajak Negara Tetangga Ramai-ramai Tinggalkan AS 2 Pengebom AS Dekati Perbatasan Rusia, Putin Kirim Jet Tempur Awas! KPK Pelototi Bisnis Batu Bara Hingga Impor Pangan Soal Libur Idul Adha 3 Hari, Ini Kata Wapres Ma'ruf Amin.